Developer Manual/Super IO
The wiki is being retired!
Documentation is now handled by the same processes we use for code: Add something to the Documentation/ directory in the coreboot repo, and it will be rendered to https://doc.coreboot.org/. Contributions welcome!
The Super I/O is a chip found on most of today's mainboards which is — among other things — responsible for the serial ports of the mainboard (e.g. COM1, COM2). This chip is usually the first thing you'll want to support, as it's required to get serial debugging output from the mainboard (via a null-modem cable and the proper software, e.g. minicom or CuteCom).
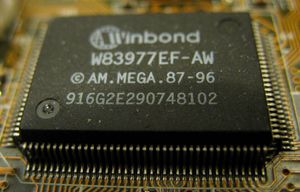
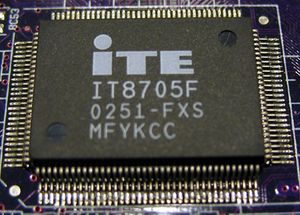
The steps for adding support for a new Super I/O chip are:
- Add a directory src/superio/vendor/device (e.g. src/superio/winbond/w83627ehg).
- In that directory, add a file device_early_serial.c (e.g. w83627ehg_early_serial.c). This file will be responsible to setup a serial port on the mainboard so that you can get serial debugging output. This will work even before the RAM is initialized, thus is useful/required for debugging the RAM initialization process.
- In this file you now declare a function device_enable_serial() which enables the requested serial port. Example:
static void w83627ehg_enable_serial(device_t dev, unsigned int iobase) { pnp_enter_ext_func_mode(dev); pnp_set_logical_device(dev); pnp_set_enable(dev, 0); pnp_set_iobase(dev, PNP_IDX_IO0, iobase); pnp_set_enable(dev, 1); pnp_exit_ext_func_mode(dev); }
- Mainboards which have this Super I/O chip, will call this function in their romstage.c file. Example:
#include "superio/winbond/w83627ehg/w83627ehg_early_serial.c" [...] #define SERIAL_DEV PNP_DEV(0x2e, W83627EHG_SP1) [...] w83627ehg_enable_dev(SERIAL_DEV, TTYS0_BASE); uart_init(); console_init();
- Whether the Super I/O is at config address 0x2e (the usual case) or 0x4e (or some other address) is mainboard-dependent. You can find out the address by running superiotool.
superio.c
While writing the superio.c part of the Super I/O bring up you will encounter the data structure static struct pnp_info pnp_dev_info[] = {} to prepare. The following information may help to understand the LDN mask pairing:
/* * io_info contains the mask 0x07f8. Given 16 register, each 8 bits wide of a * logical device we need a mask of the following form: * * MSB LSB * v v * 0x[15..11][10..3][2..0] * ------ ^^^^^ ^^^^ * null | | * | +------ Register index * | * +------------- Compare against base address and * asserts a chip_select on match. * * i.e., 0x07F8 = [00000][11111111][000] * */
Virtual logical devices
Some Super I/Os use register 0x30 of one logical device number (LDN) for more than one function enable. For example, it can be used to enable some GPIOs, GAME, MIDI etc. To overcome this issue a concept of virtual LDN has been introduced. Virtual LDNs can be used in coreboot to map the enable bit position in register 0x30 to virtual LDN, which will just enable the functionality map to that bit.
Original LDN always just switch on or off bit0 of register 0x30. Virtual LDN is created as follows. Low [7:0] bits are used to describe the original LDN. High [10:8] bits select the position of the bit enable in the register 0x30.
If LDN is 0x7 it will handle bit0 of register 0x30. If the (virtual) LDN is 0x107 it will handle bit1 of same register etc.